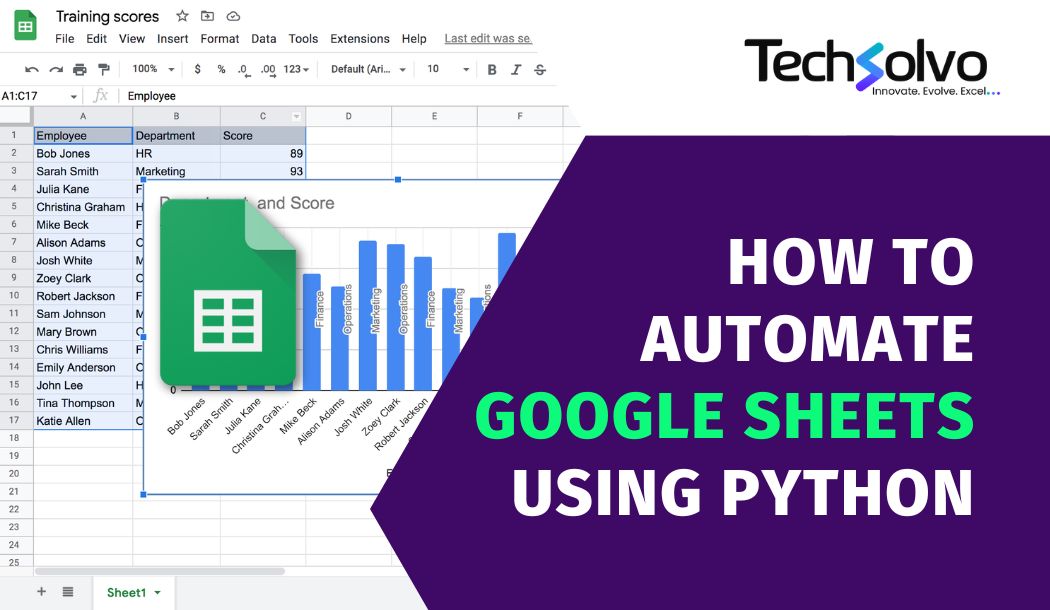
Introduction to Automation and Google Sheets
In today's fast-paced world, efficiency is key to staying ahead. Automation plays a pivotal role in streamlining tasks, freeing up valuable time, and reducing human error. By automating repetitive and time-consuming processes, individuals and businesses can focus on more strategic endeavors, leading to increased productivity and innovation.
One of the most widely used tools for data management and collaboration is Google Sheets. As part of Google's suite of cloud-based productivity applications, Google Sheets offers a powerful platform for creating, editing, and sharing spreadsheets online. Its popularity stems from several key features:
-
Accessibility: Google Sheets is accessible from any device with an internet connection, allowing users to work collaboratively in real-time, regardless of their location. This accessibility promotes seamless collaboration among teams, whether they are in the same office or spread across the globe.
-
Real-time Collaboration: Multiple users can work on the same spreadsheet simultaneously, seeing each other's changes in real-time. This fosters collaboration and eliminates the need for back-and-forth email exchanges or version control issues commonly encountered with traditional spreadsheet software.
-
Integration with Google Workspace: Google Sheets seamlessly integrates with other Google Workspace applications such as Google Docs, Google Slides, and Gmail. This integration facilitates efficient workflows, enabling users to import data from Gmail, generate reports in Google Docs, and create presentations in Google Slides directly from Google Sheets.
-
Scalability: Google Sheets can handle large datasets with ease, making it suitable for a wide range of use cases, from simple task tracking to complex financial modeling. Its cloud-based architecture ensures that users are not limited by hardware constraints and can scale their operations as needed.
-
Automation Capabilities: Google Sheets offers built-in automation features such as macros and scripts, allowing users to automate repetitive tasks and streamline workflows. This capability is particularly valuable for tasks like data import/export, data validation, and report generation, enabling users to save time and reduce errors.
In this blog post, we'll explore how to harness the power of automation using Python to interact with Google Sheets, unlocking new possibilities for efficiency and productivity in your workflows.
Setting Up Environment
Setting up your environment to interact with Google Sheets using Python involves installing the necessary libraries and configuring API credentials. Below is a step-by-step guide to help you get started:
1. Install Python:
- If you haven't already, download and install Python from the official website: https://www.python.org/downloads/.
- Follow the installation instructions for your operating system.
2. Install Required Libraries:
- Open a terminal or command prompt.
- Use pip, Python's package installer, to install the required libraries:
pip install gspread oauth2client
- These libraries will enable you to interact with Google Sheets and handle authentication.
3. Set Up Google Cloud Platform Project:
- Go to the Google Cloud Console: https://console.cloud.google.com/.
- If you don't have a Google account, you'll need to create one.
- Create a new project by clicking on the project dropdown menu at the top of the page and selecting "New Project". Give your project a name and click "Create".
- Once your project is created, select it from the project dropdown menu.
4. Enable Google Sheets API:
- In the Cloud Console, navigate to the "APIs & Services" > "Library" page.
- Search for "Google Sheets API" and click on it.
- Click the "Enable" button to enable the API for your project.
5. Create Service Account and Obtain Credentials:
- Navigate to the "APIs & Services" > "Credentials" page.
- Click on "Create credentials" and select "Service account".
- Enter a name for your service account, choose a role (e.g., Project > Editor), and click "Continue".
- Skip the optional steps and click "Done" to create the service account.
- Find the newly created service account in the list and click on the pencil icon to edit it.
- Click on "Add Key" > "Create new key".
- Choose the key type as JSON and click "Create". This will download a JSON file containing your credentials.
6. Authenticate with Google Sheets API:
- Place the downloaded JSON file in your project directory.
- In your Python script, use the credentials from the JSON file to authenticate with the Google Sheets API.
Here's a simple Python code snippet to authenticate and access a Google Sheet:
import gspread
from oauth2client.service_account import ServiceAccountCredentials
# Define scope and credentials
scope = ['https://spreadsheets.google.com/feeds', 'https://www.googleapis.com/auth/drive']
creds = ServiceAccountCredentials.from_json_keyfile_name('your_credentials_file.json', scope)
# Authenticate with Google Sheets API
client = gspread.authorize(creds)
# Access Google Sheet by its URL
sheet = client.open_by_url('your_sheet_url')
# Access specific worksheet
worksheet = sheet.get_worksheet(0)
# Now you can read, write, or manipulate data in the worksheet
By following these steps, you'll have set up your environment to interact with Google Sheets using Python and obtained the necessary credentials to authenticate with the Google Sheets API. You're now ready to start automating tasks and manipulating data in Google Sheets programmatically.
Accessing Google Sheets with Python:
To connect to a Google Sheet using Python, you can use the gspread library, which provides a simple interface for accessing Google Sheets. Here's how you can open, read, write, and update data in a Google Sheet programmatically:
import gspread
from oauth2client.service_account import ServiceAccountCredentials
# Define scope and credentials
scope = ['https://spreadsheets.google.com/feeds', 'https://www.googleapis.com/auth/drive']
creds = ServiceAccountCredentials.from_json_keyfile_name('your_credentials_file.json', scope)
# Authenticate with Google Sheets API
client = gspread.authorize(creds)
# Access Google Sheet by its URL
sheet = client.open_by_url('your_sheet_url')
# Access specific worksheet
worksheet = sheet.get_worksheet(0)
# Read data from a specific range
data = worksheet.get_all_values()
print("Data from the Google Sheet:")
print(data)
# Write data to a specific cell
worksheet.update_cell(1, 1, 'New Value')
# Update multiple cells at once
cell_list = worksheet.range('A2:B2')
for cell in cell_list:
cell.value = 'New Value'
worksheet.update_cells(cell_list)
# Append new row to the worksheet
new_row = ['Value1', 'Value2', 'Value3']
worksheet.append_row(new_row)
Automating Tasks
Automating tasks in Google Sheets using Python can significantly enhance productivity. Here's a simple example demonstrating how to automate data import/export, formatting, and calculations:
import gspread
from oauth2client.service_account import ServiceAccountCredentials
# Authenticate with Google Sheets API (same as above)
# Access Google Sheet and specific worksheet
sheet = client.open_by_url('your_sheet_url')
worksheet = sheet.get_worksheet(0)
# Data Import/Export
# Export data to a CSV file
data = worksheet.get_all_values()
with open('data.csv', 'w') as file:
for row in data:
file.write(','.join(row) + '\n')
# Import data from a CSV file
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
data = list(reader)
worksheet.update('A1', data)
# Formatting
# Apply bold formatting to a range of cells
bold_format = {'textFormat': {'bold': True}}
worksheet.format('A1:B1', bold_format)
# Calculations
# Perform a simple calculation and write the result to a cell
worksheet.update_cell(2, 1, '=SUM(A2:A5)')
Handling Advanced Operations
To handle advanced operations such as filtering data, sorting, and performing complex calculations efficiently, you can leverage Python libraries like pandas. Here's an example:
import pandas as pd
# Read data from Google Sheet into a pandas DataFrame
data = worksheet.get_all_records()
df = pd.DataFrame(data)
# Filtering data
filtered_data = df[df['Column1'] > 10]
# Sorting data
sorted_data = df.sort_values(by='Column2', ascending=False)
# Performing complex calculations
df['NewColumn'] = df['Column1'] * df['Column2']
# Writing back to Google Sheet
worksheet.clear()
for i, row in enumerate(df.values):
worksheet.insert_row(list(row), index=i+1)
This example demonstrates how you can leverage pandas to perform advanced operations efficiently and then write the results back to the Google Sheet. By using Python libraries like pandas, you can handle large datasets and complex operations with ease, thereby enhancing productivity and efficiency.
Insights
To properly understand the things that are prevalent in the industries, keeping up-to-date with the news is crucial. Take a look at some of our expertly created blogs, based on full-scale research and statistics on current market conditions.
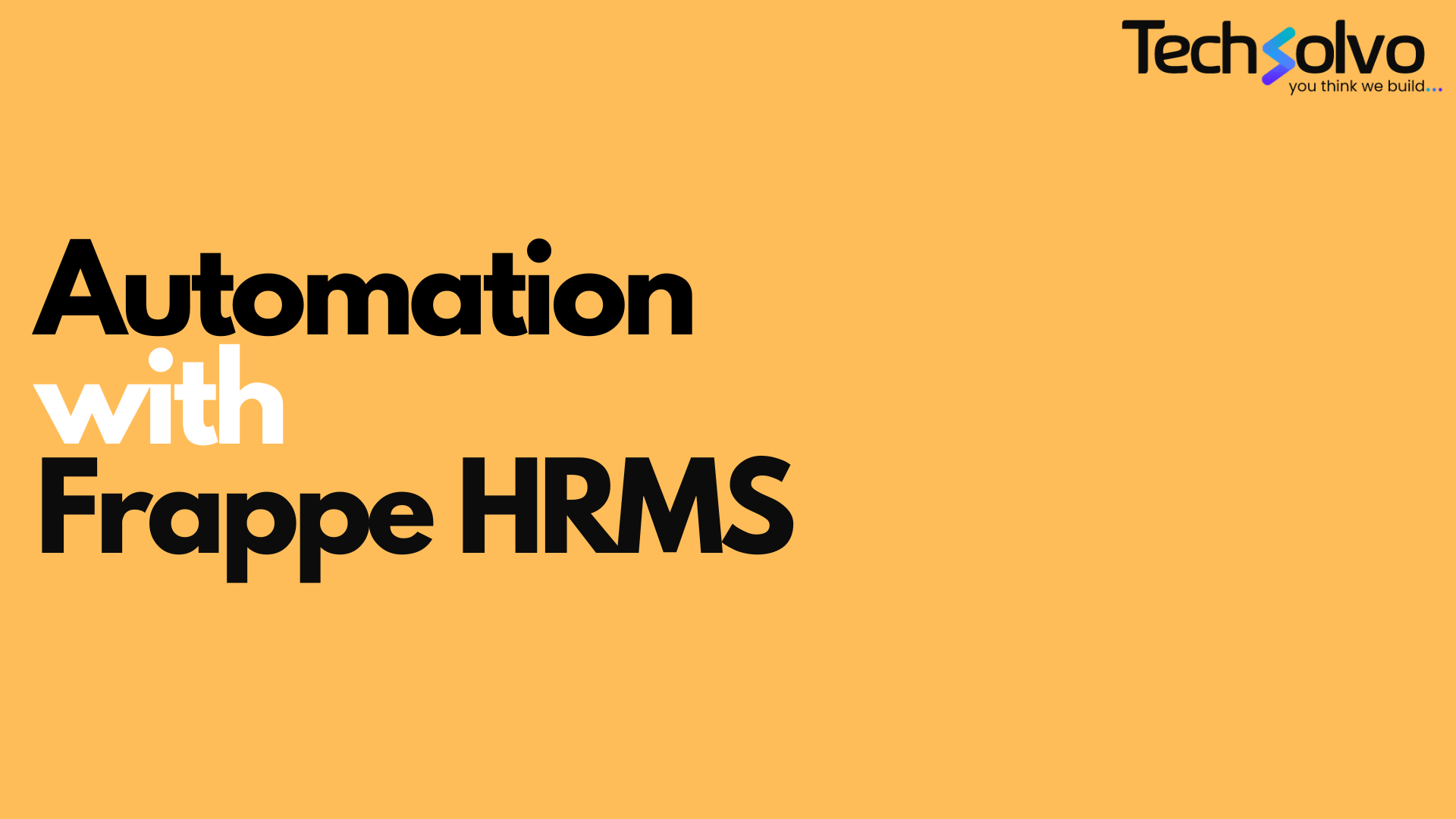
Automation in Human Resource Management with Frappe HRMS
Discover how HR automation with Frappe HRMS can streamline HR operations, enhance employe…
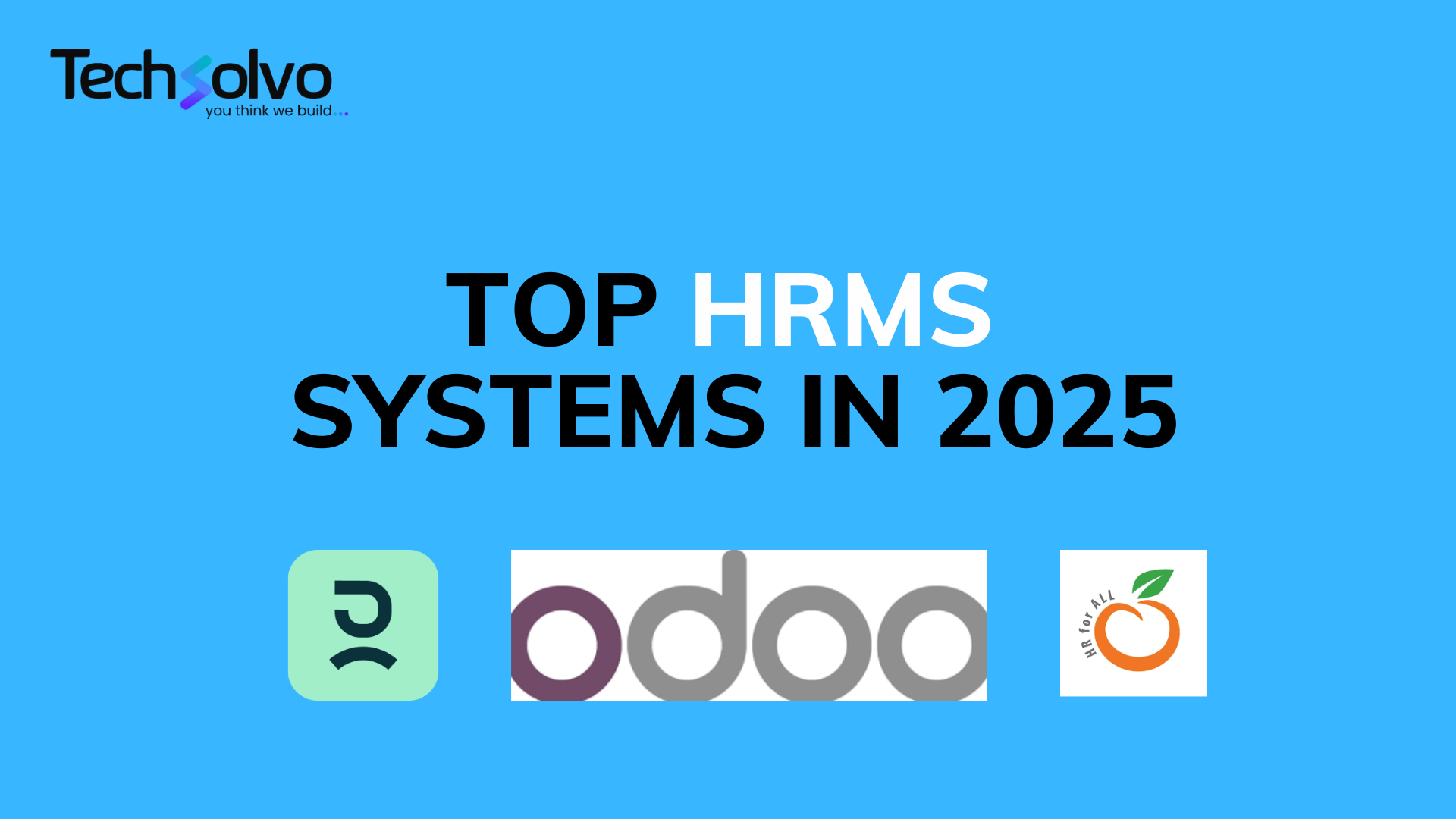
Top Open-Source HRMS Systems in 2025: The Best Free HR Software for Businesses
Discover the best open-source HRMS systems in 2025! Explore top HR software like Frappe H…
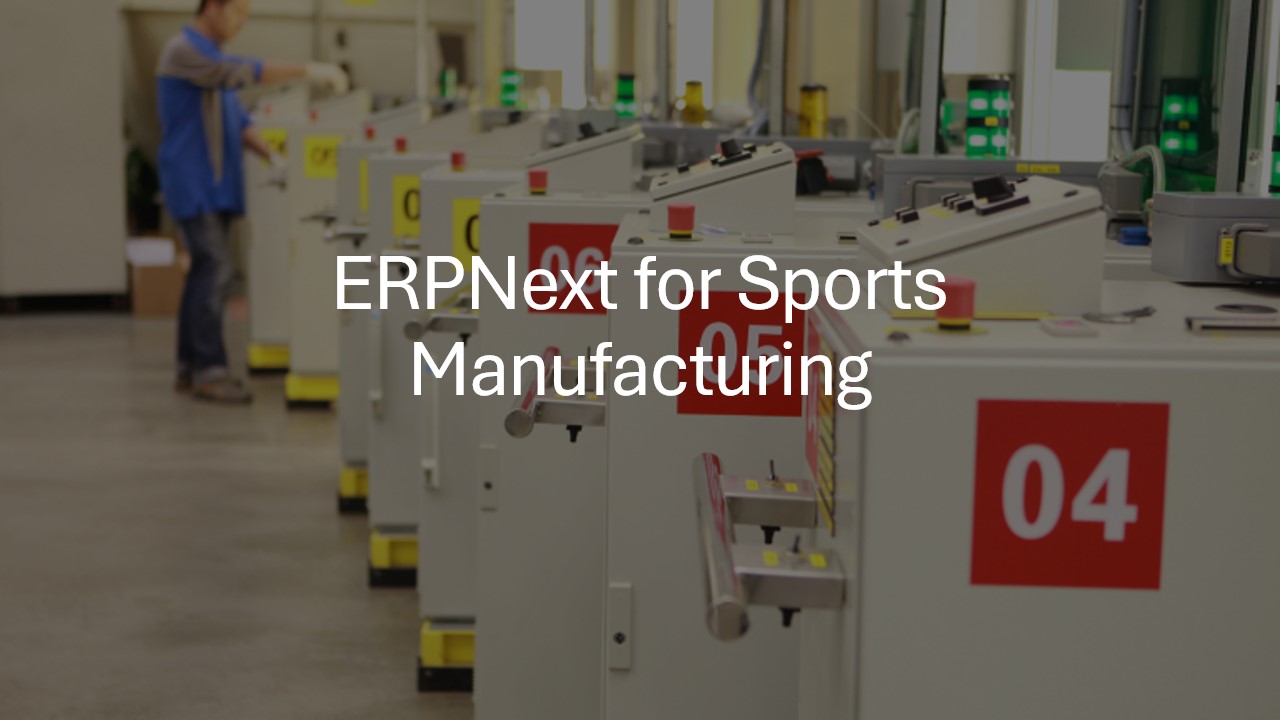
ERPNext for Sports Manufacturing: Revolutionizing Efficiency and Growth
Discover how ERPNext empowers sports equipment manufacturers to streamline operations, ma…